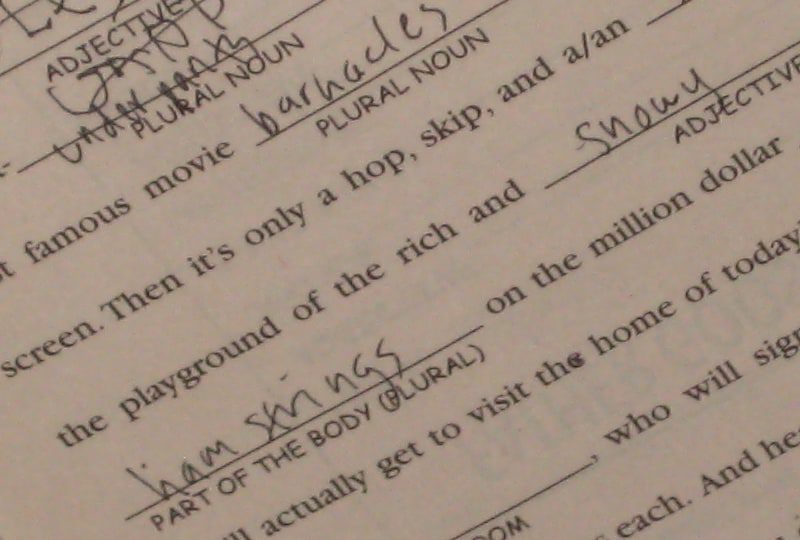
Joe Goldberg, “Mad Libs“ February 13, 2010 via Flickr, Creative Commons Attribution
It’s amazing what you can do with just a few programming concepts. What if I told you that by the time you’re done reading this post that you’ll be able to create your own version of a Mad Libs game?
Have you ever played Mad Libs? I have very fond memories of playing them with my brother on long road trips. The way it works is one person plays the role of the prompter, who has a story with very well defined fill-in-the-blanks that require parts of speech like nouns, verbs, adjectives and adverbs. The prompter asks the player or players for a word that completes a blank. After all the blanks are filled, the prompter reads the story back with the player values inserted. Hilarity ensues.
In order for us to recreate that game, we’ll need to be able to:
- Prompt the player for parts of speech
- Receive and store the player’s answers
- Output each answer into the proper blanks of the story
Oh no! Did I just automate my brother out of a job?! I’m sure I’ll find some other uses for him.
Before we get started, I need to make sure that you’ve done something. Have you completed your “Hello, World” rite of passage? Just about every tutorial you ever take is going to require you to output that phrase to your screen. If you haven’t completed this initial quest yet, I wrote up a post, How to Learn Python that’ll walk you through your initiation ceremony.
You’ll end up with a one-liner like this:
print("Hello, World")
This can be read as call the function named print and pass it the string value “Hello, World”. A function is a named group of instructions. You can use it to perform those actions whenever you want to. This is done by calling, or executing, the function. You call a function by placing opening and closing parenthesis after the function name. Functions may accept arguments, or values.
The print function that we called here got passed the value of “Hello, World”. That is a string. It was created by surrounding text in quotation marks. We just created that string and passed it into the print function, and the print function outputted our value.
After we created it, that string got thrown away. Just like my lunch did today. Last night, I cooked some tacos for dinner and stored the rest of them in a container. I put these leftovers in the office refrigerator but didn’t label my container. Sure enough, someone thought they were old and just threw them away. 🙁 I should’ve labelled it.
Our office refrigerator rules are similar to Python’s. If you want something to stick around, you have to label it.
So we know that we can create a new string by surrounding our characters with quotes:
"Craig"
This is known as a string literal. That line of code creates a string with my name in it — but because it’s not labelled, it gets thrown away.
So let’s apply a label:
first_name = "Craig"
Now that we’ve created a new label, or name, that we can use it in our program. Our label, first_name, is also referred to as a variable. Our first_name variable will give us access to the object that we labelled.
So we could print our string like this:
print(first_name)
# Outputs: Craig
An important thing to notice here is that there aren’t quotes around first_name. This line can be read as call the print function passing in the value of the variable named first_name.
Thus far, we’ve only used the print function with a single argument. But in fact, you can pass multiple arguments to it, and what happens is that it prints each value out on the same line but separated by spaces. To pass multiple arguments to a function, you just separate each value you want to pass with a comma.
Here’s an example of multiple arguments at play:
print("You", "can", "actually", "pass", "multiple", "values to the", "print function")
# Outputs: You can actually pass multiple values to the print function
Note how each of the strings are joined together and are separated by spaces. With this new information in hand, what do you say we make our original Hello World example a little more personalized:
print("Hello", first_name)
# Outputs: Hello Craig
Well, this feels a little rude. I’m just saying hello to myself. I’d like it to say hello to you, but I don’t know your name. Awkward. I could actually ask you for it.
What we’ve been doing so far is all output, right? We’ve been outputting to the screen, but now I want to take input from our user. In this case, the user is you.
I’ve embedded an editor and a python shell from the wonderful site REPL.it. Just press the play button to run our script:
Aww, that feels better. Nice to meet you. See how the code used a new function named input? Input accepts a parameter, which is the prompt we want to display to our user. That’s where we passed in the string “What is your first name? ” Note that I left a few spaces because right after the prompt is where the cursor will sit waiting for the user to press the Enter key.
So the input function waits for an answer, and once it receives it, the new string is returned. A new string with your name in it was created, and then we applied the label of first_name. We then printed out using our multiple arguments provided to the print function trick we picked up.
Now, what were we doing … oh yeah, Mad Libs! Let’s review our plan:
- Prompt the player for parts of speech
We can do this now! Just use that new input function we just added to our bag of tricks.
- Receive and store answer from the player
And we can store the values in variables! We’ll just label the responses clearly and then we can use them later.
- Output each answer into the proper blanks of the story
Oooh, and we can just use the print function to print out the story structure, and then make each of our variables fill in the blanks!
So here’s my first attempt:
Does it make sense? Isn’t that cool?! We were able to build a program just by picking up some foundational programming skills. Feel free to dork around with that and tweak it however you like — just like those new Python skills you just picked up, it’s all yours.
I hope you had fun and are hooked on learning. If you enjoyed this and would like to continue, please join me in the Beginning Python Track on Treehouse. We’ll keep building up your Python skills, and nothing is going to stop you from creating whatever you can dream up. I’ll invite my brother too, he’s been pretty bored ever since we automated him out of his Mad Libs job.
print("Nice meeting you,", first_name)
print("Looking forward to hanging out more with you")
Interested in a guided curriculum that leads you from Python beginner to job-ready developer? Check out our Techdegrees.
Free 7-day Techdegree Trial