In software development, every coder has unique quirks and preferences that make their coding style distinct. But these differing approaches to naming conventions, indentation and spacing, error handling, and more, can make team collaboration challenging. That’s before you even consider unintuitive, complex code. This is where code comments come in.
If you want to know how to write good comments in code, it starts with them being meaningful. They should explain a developer’s reasoning and logic, acting as a guide that allows every team member to understand, maintain, and modify the code—no matter when they join a project.
In this post, we’ll outline the best practices and pitfalls to avoid to promote effective knowledge sharing and enhance collaboration among developers.
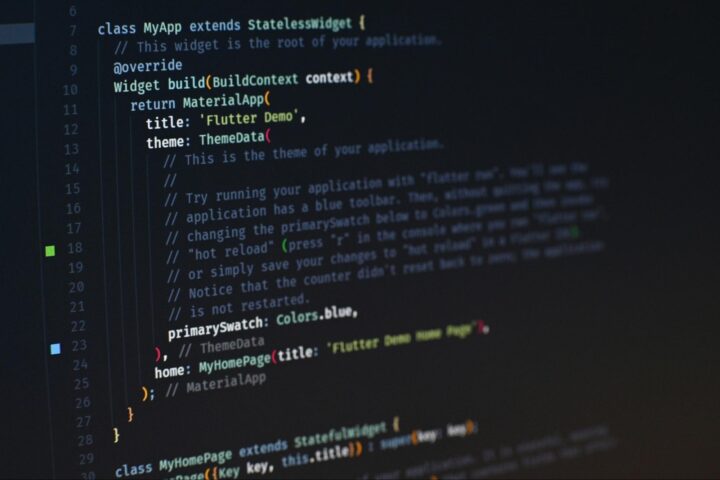
Contents
What Are Code Comments?
Code comments are human-readable annotations developers include within sections of source code. They add context to the code and make it easier to understand. Comments are marked with specific characters to avoid being evaluated by the program and, therefore, don’t affect the code’s behavior.
Comments should:
- Be written in natural language, using accessible English statements.
- Explain the code’s purpose and functionality.
- Describe any algorithms used to achieve this.
- Highlight other useful implementation details.
- Flag up potential issues or pieces of code that require particular attention.
Types of Code Comments
Comments typically use one of two types of syntax based on the length of source code to be annotated.
The first is called a single-line/inline comment, which applies to a single line in the program. The second is a multi-line/block comment and describes a paragraph of text. Let’s look at each in more detail.
Single-line/Inline Comments
A single-line comment is placed in the body of the line of code. It describes and provides a very brief explanation of what’s going on. Use single-line comments to help team members get up to speed with specific parts of code that are complex or not intuitive.
Be aware that commenting on every single line of code will have the exact opposite effect, rendering code less readable.
In the programming language Java, use // before your comment for a single-line comment. The program will ignore everything from // to the end of the line.
Multi-line/Block Comments
Block comments provide detailed explanations for a section of code or complex function. They offer valuable context, enabling team members to understand the overall purpose and logic of a code segment to enhance collaboration and ensure smoother project development.
In Java, use the following /* text */ for a block comment.
How Do Comments Boost Collaboration?
It’s important to remember that you aren’t only writing code for your computer to understand and process. It’s also a reference for yourself and other team members who you’re collaborating with.
Comments clarify data collection methods used in functions, aiding comprehension and teamwork. For example, a comment like “This function aggregates customer data from multiple sources using our approved data collection methods, ensuring consistency and reliability for marketing analysis” offers clear insights into the data processes. This is beneficial for code maintenance, training new developers, and enhancing team collaboration.
With more companies using diversity recruiting platforms and searching for remote talent around the world, there’s every chance that others on your team have differing skills and experience.
Even if your code is well-written, there’s no guarantee that colleagues won’t find it confusing or ambiguous.
For broader project insights, tools like Microsoft Power BI can be utilized to track and visualize coding metrics or team productivity, which can be discussed within code comments to further enhance understanding across the team.
When it comes to large projects with distributed teams, code comments also serve as a means of communication, helping developers to work together seamlessly.
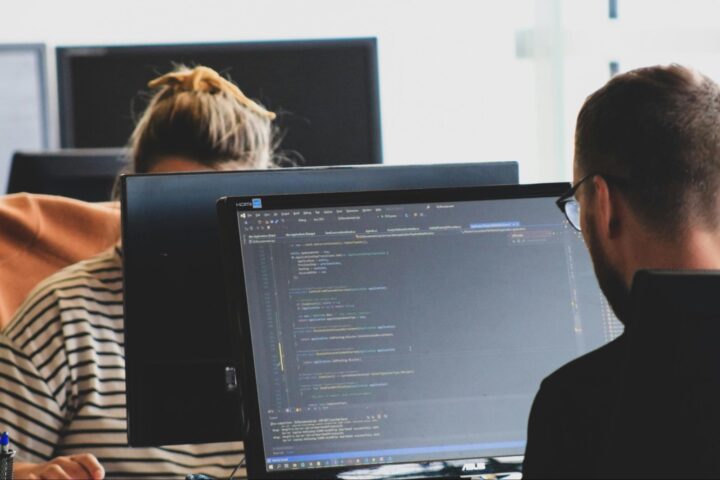
Let’s say a team of developers is building a virtual phone system that allows users to make and manage customer calls over the internet. One frontend developer might work on coding for the system’s interactive dashboard while another builds the visual call flow builder. A third must create forms for setting up auto-attendants and call routing rules built into the call flow.
By engaging with the code comments left by the original developer, the next should be able to understand and pick up on the existing implementation quickly and make the necessary changes without interfering with the code’s functionality.
Best Practices for Writing Good Code Comments
We’ve established that providing effective code comments is critical to maintaining a readable, accessible, and maintainable codebase. Follow these key best practices so you can do just that while promoting collaboration within your development team.
Give More Context
Use your comment to clearly explain the why of your code—that is, the context, logic, and reasoning behind it—rather than simply what it does. This makes it easier for future developers to understand the intent of original developers, and means they can continue to maintain, update, or improve the code with that in mind.
Let’s say you’re building an HR platform and your app relies on efficient data retrieval to carry out high-volume hiring. Instead of providing an unclear code comment like “This function formats a date,” explain the reasoning behind the code and why it’s important.
A comment like “This function formats a date to the ‘YYYY-MM-DD’ format to ensure consistency with the database storage requirements” immediately provides developers with a more readable, maintainable, and modifiable codebase to collaborate on.
Be Clear and Concise
Keep comments short, focused, and clear to maintain readability. Comments shouldn’t state the obvious but be restricted to the most valuable information.
Use simple, descriptive language, avoiding complex terminology and jargon that could confuse team members. Make sure your comments don’t repeat the code itself or any other extraneous details that don’t add value.
Here’s an overly wordy, complex comment that contains unnecessary details:
“This function, utilizing a highly optimized algorithm, iterates over the entire list of user objects to compute the total number of active users, ensuring that the computational complexity remains linear, O(n). It starts by initializing a counter to zero, then it goes through each user in the list one by one, checking if the user is active, and if so, it increments the counter by one. Finally, it returns the counter, which represents the total number of active users.”
A comment like this will undoubtedly cause developers to make errors. They’ll struggle to grasp the code’s purpose and functionality. What’s more, they’ll likely spend significant time deciphering the comment, causing team members to feel alienated and hindering teamwork.
Here’s a more direct, concise alternative:
“This function counts the number of active users in a list using a linear scan to monitor user engagement.”
This comment is more accessible to team members of all levels, improving coding confidence and enhancing collaboration. It’s direct and clearly explains the function and why it’s useful without overdoing it.
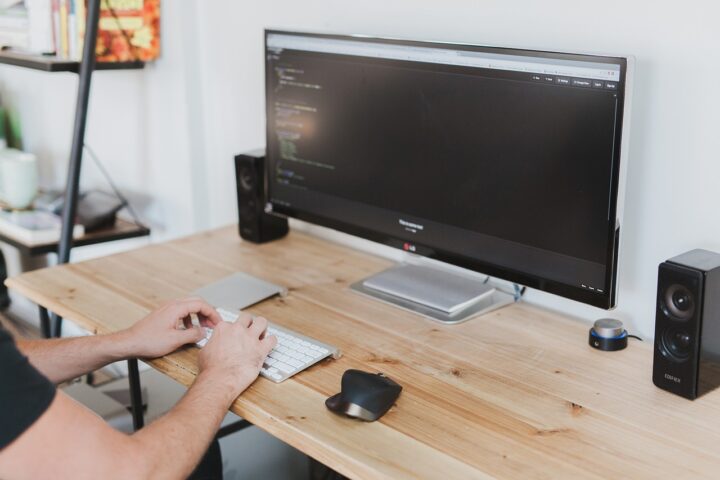
Keep Your Comments Up to Date
If you moved from California to New York, you’d update your number to a 646 area code. Similarly, you have to maintain and update code comments to reflect any changes in your codebase. It’s key to maintaining the accuracy and relevance of your comments.
Outdated comments cause confusion. They’re open to misinterpretation and errors and compromise collaboration. In scenarios where developers might use voice-to-text tools to quickly document their code, ensuring transcription accuracy is crucial to avoid miscommunications.
Brief your team to also update corresponding comments when they modify code. Review comments regularly and refactor them to weed out redundant ones.
Be Consistent with Your Commenting Style
Maintaining a consistent commenting style throughout your codebase boosts code readability and reduces misunderstandings.
To prioritize consistency, make sure you:
- Use the same formatting approach, such as spacing and line length. This ensures comments are easy to read and developers can distinguish between comments and code.
- Standardize the use of terminology across your codebase.
- Document the conditions/circumstances you’ve taken as true (assumptions) and the requirements you’ve met for your code to be executed successfully (preconditions). This will help developers grasp code context.
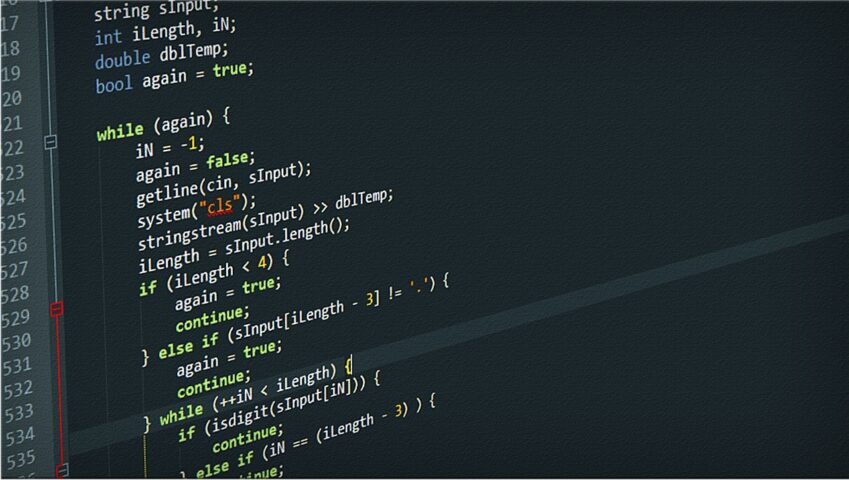
Clarify Complex Code
Whether developers have just finished their tech degree or have been in the industry for years, non-obvious code can be difficult to understand. Use comments to clarify complex logic and explain why code is written the way it is.
For example, if you’re coding a function to calculate a discount price and ensure that the final price isn’t negative, insert a comment explaining why this check is necessary so colleagues understand the edge case it handles. Such a comment might look like this: “This handles cases where the discount is greater than the price”.
Similarly, when dealing with domain name validation or routing logic in a web application, clear comments can help other developers understand how domain names are processed and avoid potential misconfigurations.
Common Pitfalls to Avoid for Better Collaboration
We’ve established how to write meaningful comments that foster collaboration. But let’s look at the most frequent mistakes developers make that often end up alienating team members and causing mistakes.
Over-Commenting and Under-Commenting
Striking the right balance with the number of comments you include within your code is critical. Over-commenting creates clutter, which obscures logic and purpose, is hard to read and maintain, and stymies collaboration.
On the other hand, under-commenting leaves significant gaps in understanding, especially for less experienced developers and new team members.
Provide enough information to explain the context and functionality of code, particularly complex or non-obvious sections, without overwhelming the reader.
A good rule of thumb is to make no more than one comment per ten lines of code.
Making up for Bad Code with Good Comments
Comments shouldn’t be used as a crutch to explain confusing or overly complex code. Relying on comments to explain bad code makes the codebase harder to understand and maintain in the long run.
Prioritize writing clean, readable code first, then use comments to enhance clarity where necessary.
Write Good Comments and Collaboration Will Follow
Whether you’re embarking on your first 100 days of code or are a seasoned developer, understanding the value of writing clear and informative comments is imperative for those who strive to maintain a readable and modifiable codebase.
Good comments are clear, up-to-date, consistent, and helpful. They enable developers to understand each other’s work and contribute to projects effortlessly. As a result, all team members feel empowered to work together in producing higher code quality and, ultimately, more successful project development.
Land Your Dream Front End Web Developer Job in 2024!
Learn to code with Treehouse Techdegree’s curated curriculum full of real-world projects and alongside incredible student support. Build your portfolio. Get certified. Land your dream job in tech. Sign up for a free, 7-day trial today!
Start a Free Trial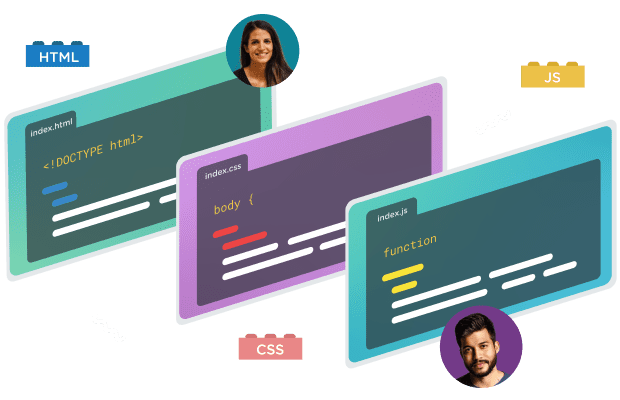